Dart Programming - Unit 测试
单元测试涉及测试应用程序的每个单元。 它可以帮助开发人员在不运行整个复杂应用程序的情况下测试小功能。
名为“test”的Dart external library提供了编写和运行单元测试的标准方法。
Dart_programming单元测试涉及以下步骤 -
Step 1: Installing the "test" package
要在当前项目中安装第三方软件包,您需要pubspec.yaml文件。 要安装test packages ,首先在pubspec.yaml文件中进行以下输入 -
dependencies:
test:
输入后,右键单击pubspec.yaml文件并获取依赖项。 它将安装"test"包。 下面给出了WebStorm编辑器中相同的屏幕截图。
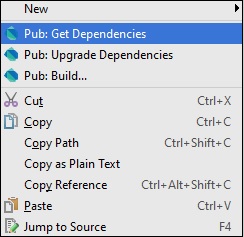
包也可以command line安装。 在终端中键入以下内容 -
pub get
Step 2: Importing the "test" package
import "package:test/test.dart";
Step 3 Writing Tests
使用顶级函数test()指定test() ,而使用expect()函数进行test assertions 。 要使用这些方法,应将它们安装为pub依赖项。
语法 (Syntax)
test("Description of the test ", () {
expect(actualValue , matchingValue)
});
group()函数可用于对测试进行分组。 每个组的描述都添加到其测试描述的开头。
语法 (Syntax)
group("some_Group_Name", () {
test("test_name_1", () {
expect(actual, equals(exptected));
});
test("test_name_2", () {
expect(actual, equals(expected));
});
})
例1:通过测试
以下示例定义方法Add() 。 此方法采用两个整数值并返回表示sum的整数。 要测试这个add()方法 -
Step 1 - 导入test包,如下所示。
Step 2 - 使用test()函数定义测试。 这里, test()函数使用expect()函数来强制执行断言。
import 'package:test/test.dart';
// Import the test package
int Add(int x,int y)
// Function to be tested {
return x+y;
}
void main() {
// Define the test
test("test to check add method",(){
// Arrange
var expected = 30;
// Act
var actual = Add(10,20);
// Asset
expect(actual,expected);
});
}
它应该产生以下output -
00:00 +0: test to check add method
00:00 +1: All tests passed!
示例2:失败测试
下面定义的subtract()方法存在逻辑错误。 以下test验证相同。
import 'package:test/test.dart';
int Add(int x,int y){
return x+y;
}
int Sub(int x,int y){
return x-y-1;
}
void main(){
test('test to check sub',(){
var expected = 10;
// Arrange
var actual = Sub(30,20);
// Act
expect(actual,expected);
// Assert
});
test("test to check add method",(){
var expected = 30;
// Arrange
var actual = Add(10,20);
// Act
expect(actual,expected);
// Asset
});
}
Output - 函数add()的测试用例通过,但subtract()的测试失败,如下所示。
00:00 +0: test to check sub
00:00 +0 -1: test to check sub
Expected: <10>
Actual: <9>
package:test expect
bin\Test123.dart 18:5 main.<fn>
00:00 +0 -1: test to check add method
00:00 +1 -1: Some tests failed.
Unhandled exception:
Dummy exception to set exit code.
#0 _rootHandleUncaughtError.<anonymous closure> (dart:async/zone.dart:938)
#1 _microtaskLoop (dart:async/schedule_microtask.dart:41)
#2 _startMicrotaskLoop (dart:async/schedule_microtask.dart:50)
#3 _Timer._runTimers (dart:isolate-patch/timer_impl.dart:394)
#4 _Timer._handleMessage (dart:isolate-patch/timer_impl.dart:414)
#5 _RawReceivePortImpl._handleMessage (dart:isolate-patch/isolate_patch.dart:148)
分组测试用例
您可以对test cases进行分组,以便为测试代码添加更多含义。 如果您有许多test cases这有助于编写更清晰的代码。
在给定的代码中,我们正在为split()函数和trim函数编写测试用例。 因此,我们在逻辑上将这些测试用例分组并称之为String 。
例子 (Example)
import "package:test/test.dart";
void main() {
group("String", () {
test("test on split() method of string class", () {
var string = "foo,bar,baz";
expect(string.split(","), equals(["foo", "bar", "baz"]));
});
test("test on trim() method of string class", () {
var string = " foo ";
expect(string.trim(), equals("foo"));
});
});
}
Output - 输出将附加每个测试用例的组名称,如下所示 -
00:00 +0: String test on split() method of string class
00:00 +1: String test on trim() method of string class
00:00 +2: All tests passed